In Laravel’s Eloquent ORM, there are several types of relationships that you can define between models. These relationships allow you to easily retrieve and manipulate related data. Here are the available types of relationships in Laravel Eloquent:
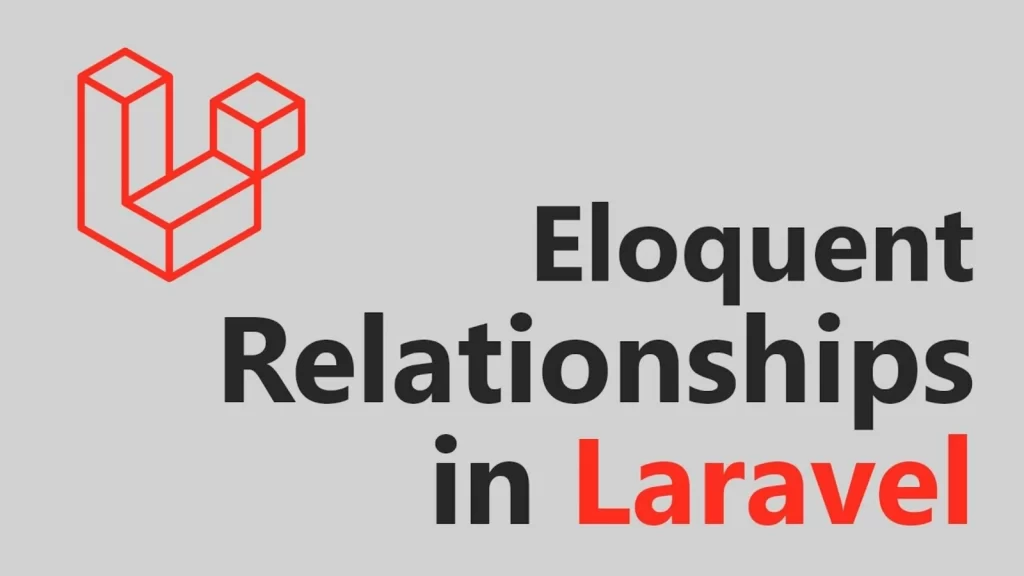
- One-to-One (1:1) Relationship:
hasOne
: Defines a one-to-one relationship where the current model has a single related model.belongsTo
: Defines an inverse of thehasOne
relationship, indicating that the current model belongs to a single related model.
- One-to-Many (1:N) Relationship:
hasMany
: Defines a one-to-many relationship where the current model has multiple related models.belongsTo
: Defines an inverse of thehasMany
relationship, indicating that the related model belongs to a single current model.
- One-to-Many (1:N) Relationship:
hasMany
: Defines a one-to-many relationship where the current model has multiple related models.belongsTo
: Defines an inverse of thehasMany
relationship, indicating that the related model belongs to a single current model.
- Many-to-Many (N:N) Relationship:
belongsToMany
: Defines a many-to-many relationship where the current model has multiple related models, and the related model also has multiple current models. This relationship requires a pivot table to store the intermediate data.
- Has-One-Through Relationship:
hasOneThrough
: Defines a one-to-one relationship through an intermediate model, allowing you to access a related model indirectly.
- Has-Many-Through Relationship:
hasManyThrough
: Defines a one-to-many relationship through an intermediate model, allowing you to access multiple related models indirectly.
- Polymorphic Relationship:
morphTo
: Defines a polymorphic relationship where the current model can belong to multiple other models.morphOne
/morphMany
: Defines the inverse ofmorphTo
, indicating that multiple models can have a polymorphic relationship with the current model.
- Polymorphic Many-to-Many Relationship:
morphToMany
: Defines a many-to-many polymorphic relationship where the current model can have multiple related models with different types.morphedByMany
: Defines the inverse ofmorphToMany
, indicating that multiple models can have a many-to-many polymorphic relationship with the current model.
These are the available types of relationships in Laravel Eloquent. By defining these relationships in your model classes, you can easily navigate and work with related data in your application.