In Laravel, a “job” refers to a unit of work or a task that can be executed asynchronously in the background. Jobs in Laravel are part of Laravel’s queueing system, which allows you to offload time-consuming or resource-intensive tasks to be processed outside of the typical request-response cycle. Jobs can include tasks such as sending emails, generating reports, processing data, or performing any other task that can benefit from asynchronous processing.
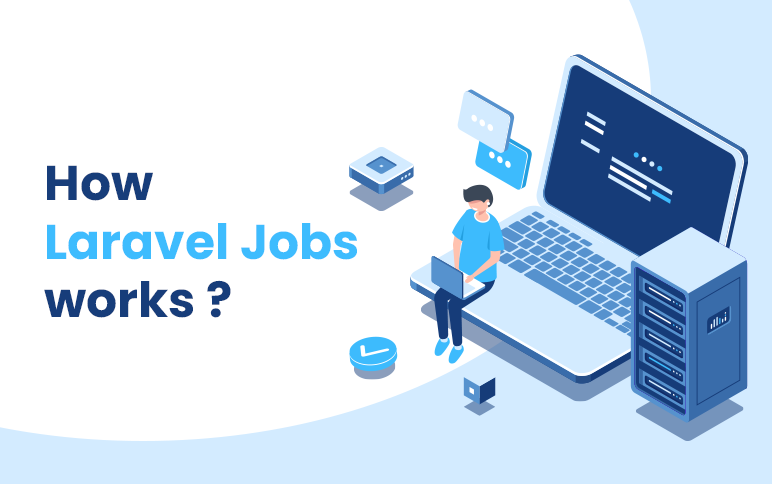
Here are some key aspects and benefits of using jobs in Laravel:
- Asynchronous Processing: By defining a job and dispatching it to a queue, Laravel handles the execution of the job in the background, separate from the user’s request. This helps improve the responsiveness and performance of your application by offloading time-consuming tasks to be processed asynchronously.
- Queueing System: Laravel provides a built-in queueing system that allows you to manage and prioritize jobs. You can configure different queues, specify queue connections and workers, and control the processing order of jobs.
- Delayed Execution: Jobs can be scheduled to run at a specific time or after a certain delay. This allows you to schedule tasks to be executed in the future, which can be useful for tasks like sending reminder emails or processing periodic reports.
- Retry and Failure Handling: Laravel’s queueing system includes robust error handling and retry mechanisms. If a job fails during processing, you can configure the system to automatically retry the job a certain number of times. You can also define custom failure handlers to handle failed jobs and log errors for further investigation.
- Scalability: Jobs in Laravel enable horizontal scalability by allowing you to distribute the workload across multiple workers or queues. This helps handle high volumes of jobs and ensures efficient resource utilization.
- Job Chaining and Dependencies: Laravel allows you to define dependencies and relationships between jobs. This means that you can specify that a job should only run after another job has completed successfully. This helps orchestrate complex workflows and ensures tasks are executed in the desired order.
- Monitoring and Reporting: Laravel provides tools and utilities for monitoring and reporting on job execution. You can track the status and progress of jobs, view job logs, and gather performance metrics to analyze and optimize your application’s background processing.
Jobs in Laravel are implemented using the `Illuminate\Contracts\Queue\ShouldQueue
` interface, which indicates that a job should be queued for asynchronous processing. By defining and dispatching jobs in Laravel, you can effectively manage and execute background tasks, freeing up your application to handle user requests more efficiently and improving overall performance.
Here are a few examples of jobs that can be implemented in Laravel:
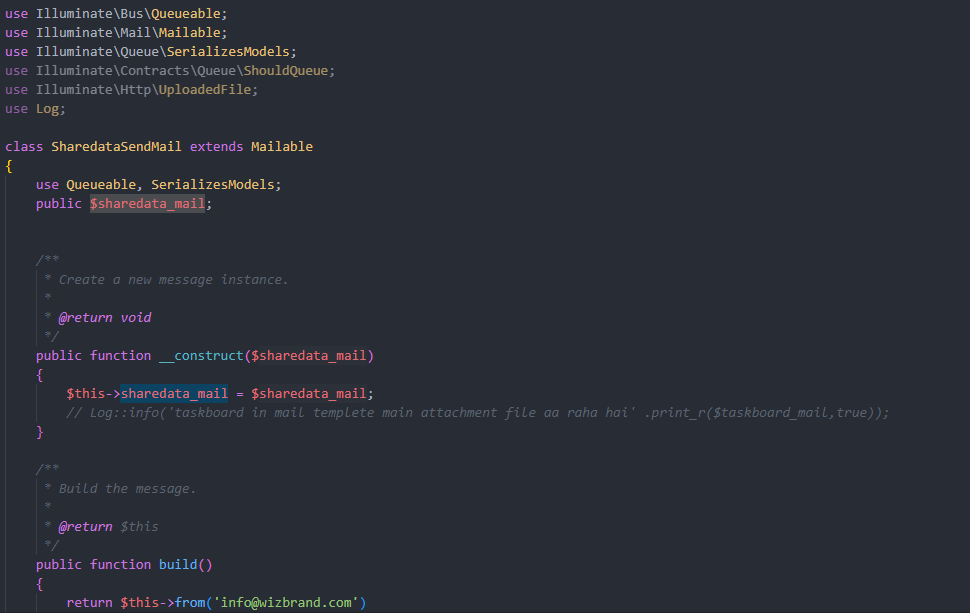
- Sending Email: You can create a job to send emails asynchronously. This can be useful for tasks like sending welcome emails to new users, notifications, or batch email sending.
- Image Processing: If you have an image-heavy application, you can create a job to process and resize images. This can include tasks such as cropping, resizing, or applying filters to images uploaded by users.
- Generating Reports: Jobs can be used to generate reports in the background. For example, you can create a job that fetches data from a database, processes it, and generates a PDF or Excel report.
- Performing Data Import/Export: If you need to import or export large datasets, you can create jobs to handle these operations asynchronously. This allows users to initiate the import or export process and continue using the application while the job processes the data in the background.
- Integrating with Third-Party APIs: Jobs can be used to interact with external APIs asynchronously. For example, you can create a job to fetch data from a third-party API and store it in your application’s database.
- Processing Payments: If you have a payment gateway integration, you can create a job to handle payment processing asynchronously. This can involve tasks like verifying payments, updating transaction records, and sending payment confirmation emails.
- Notifying Subscribers: Jobs can be used to send notifications or updates to subscribers. For instance, you can create a job that sends push notifications or updates to mobile devices when new content becomes available.
- Performing Data Cleanup: Jobs can be used for routine data cleanup tasks. For example, you can create a job that deletes expired or outdated records from the database, ensuring data integrity and efficient storage.