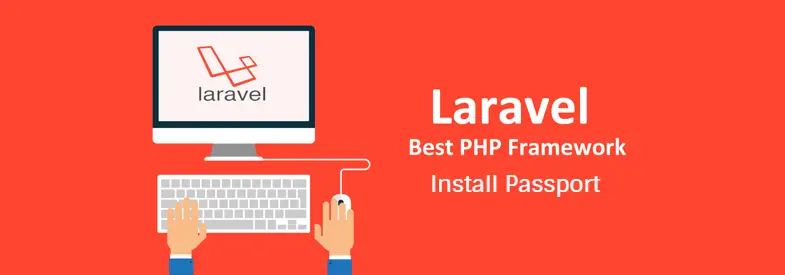
When you run the passport:install
command in Laravel Passport, it performs the following actions:
- Generates Encryption Keys: Passport uses encryption keys to sign access tokens, so running
passport:install
generates the encryption keys required for token generation and validation. The generated keys are stored in thestorage
directory of your Laravel application. - Creates Database Tables: Passport requires database tables to store access tokens, refresh tokens, and other related information. The
passport:install
command creates the necessary migration files for these tables. You need to run themigrate
command to execute the migrations and create the tables in your database. - Adds Passport Routes and Middleware: The command registers the necessary routes and middleware for the Passport in your application. These routes handle authentication, token generation, and token revocation.
- Adds Configuration Settings: Passport requires some configuration settings to work properly. The
passport:install
the command adds the necessary configuration settings to yourconfig/auth.php
file.
In summary, running passport:install
in Laravel Passport sets up the encryption keys, creates database tables, registers routes, and middleware, and adds configuration settings to enable the usage of Passport for API authentication and token management in your Laravel application.
Passport working:-
In Laravel Passport, the package provides a complete OAuth2 server implementation that allows you to authenticate and authorize access to your API endpoints. It simplifies the process of adding OAuth2 authentication to your Laravel application and allows you to issue access tokens, refresh tokens, and manage client applications.
Here’s how Laravel Passport typically works:
- Installation and Setup: First, you need to install the Laravel Passport package using Composer. Once installed, you run the
passport:install
command to set up the necessary encryption keys, database tables, routes, middleware, and configuration files. - API Authentication: Passport enables you to secure your API endpoints using OAuth2 authentication. You can define which routes or middleware should require authentication by applying the
auth:api
middleware. When a request is made to an authenticated route, Passport checks for a valid access token in the request header. - Client Applications: Passport allows you to create and manage client applications that can access your API. You can generate client application keys and secrets using the
passport:client
command. These keys and secrets are used to authenticate and authorize client applications when they request access tokens. - Access Tokens: To access protected API endpoints, client applications must obtain an access token from the OAuth2 server. Client applications can request access tokens by sending a client ID, client secret, and other required parameters to the token endpoint of your application. The OAuth2 server validates the client credentials and issues an access token.
- Token Scopes: Passport supports token scopes, which allow you to define the permissions or scopes associated with an access token. Scopes restrict the actions a client application can perform on behalf of the authenticated user. You can define scopes and assign them to client applications when requesting access tokens.
- Token Revocation and Refreshing: Passport provides mechanisms to revoke and refresh access tokens. Clients can revoke an access token to invalidate it before its expiration time. Additionally, clients can use a refresh token to obtain a new access token without requiring the user to re-enter their credentials.
- API Authorization: Passport also integrates with Laravel’s built-in authorization system. You can define access policies to control what resources or actions a user or client application can access. These policies can be enforced using Passport’s middleware and applied to your API routes.
Laravel Passport makes it easier to implement OAuth2 authentication and authorization in your Laravel application, allowing you to secure your APIs and control access to protected resources. It provides the necessary tools and features to manage clients, issue access tokens, and handle token revocation and refresh.