In Laravel’s query builder, you can use several types of joins to combine data from multiple database tables. Here are the types of joins commonly used in Laravel:
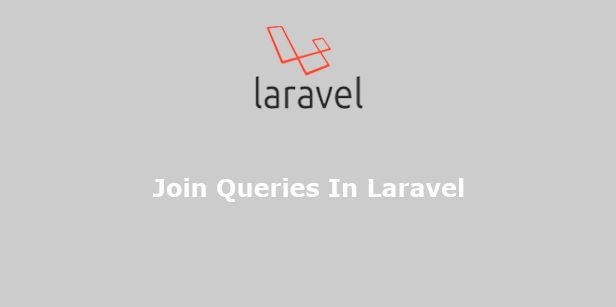
1. Inner Join:
An inner join returns only the matching records from both tables based on the specified join condition.
$query->join('table2', 'table1.column', '=', 'table2.column');
2. Left Join (Outer Join):
A left join returns all records from the left table and the matching records from the right table based on the join condition. If there are no matches in the right table, NULL values are returned.
$query->leftJoin('table2', 'table1.column', '=', 'table2.column');
3. Right Join (Outer Join):
A right join returns all records from the right table and the matching records from the left table based on the join condition. If there are no matches in the left table, NULL values are returned.
$query->rightJoin('table2', 'table1.column', '=', 'table2.column');
4. Cross Join:
A cross join returns the Cartesian product of the two tables, combining each row from the first table with every row from the second table.
$query->crossJoin('table2');
5. Joining Multiple Tables:
You can also join multiple tables by chaining multiple join methods or specifying an array of join conditions.
$query->join('table2', 'table1.column', '=', 'table2.column')
->join('table3', 'table1.column', '=', 'table3.column');
These are the commonly used join types in Laravel’s query builder. Depending on your application’s requirements, you can choose the appropriate join type to retrieve and combine data from multiple database tables.