Hello Laravel Developers,
In this post, we will explore how to use the Laravel Eloquent framework to apply a “not equal to” condition in your database queries. This example will demonstrate how to utilize Laravel’s Eloquent to filter records where a specific column does not have a certain value.
In Laravel’s Eloquent, the where
method is a powerful tool for adding conditions to your database queries. One common use case is when you want to retrieve records where a particular column is not equal to a specific value. You can achieve this by using either the ‘!=
‘operator or the ‘<>
‘ operator. Let’s delve into how to use both of these operators:
Let’s say you have a “users” table, and you want to fetch all users whose “status” column does not equal a specific value, such as “0”. Here’s a representation of a simple table with sample data:
users Table:
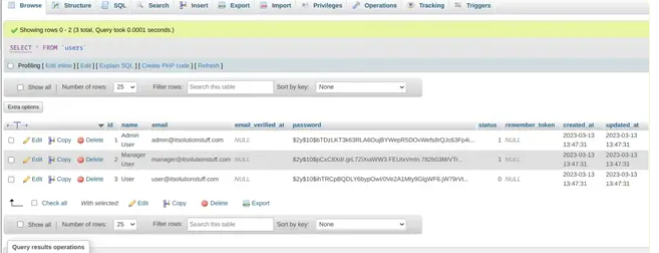
Laravel Where Not Equal To using “!=” Operator:
the simple code of UserController File.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index(Request $request)
{
$users = User::select("id", "name", "email", "status")
->where('status', '!=', '0')
->get();
dd($users);
}
}
Output:-
Array
(
[0] => Array
(
[id] => 1
[name] => Admin User
[email] => admin@cotocus.com
[status] => 1
)
[1] => Array
(
[id] => 2
[name] => Manager User
[email] => manager@cotocus.com
[status] => 1
)
)
Laravel Where Not Equal To using “<>” Operator:
the simple code of UserController File.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index(Request $request)
{
$users = User::select("id", "name", "email", "status")
->where('status', '<>', '0')
->get();
dd($users);
}
}
Output:
Array
(
[0] => Array
(
[id] => 1
[name] => Admin User
[email] => admin@cotocus.com
[status] => 1
)
[1] => Array
(
[id] => 2
[name] => Manager User
[email] => manager@cotocus.com
[status] => 1
)
)
I hope it can help you!!!!!