We will explore how to implement an infinite scroll feature with a dynamic Next button in Laravel search results. When searching for data in a web application, it’s common to display the results in multiple pages. However, instead of traditional pagination, we will implement an infinite scroll approach where additional results are loaded automatically as the user scrolls down the page. If there are no more results to display, the Next button will be hidden. This implementation will provide a smoother and more engaging user experience. Let’s get started!
Step 1:- HTML Part
<div class="pagination-buttons" style="float: left; transform: translate(-104%, 117%);">
<button id="previous_button" class="previous_button relative inline-flex items-center px-4 py-2 text-sm font-medium text-gray-500 bg-white border border-gray-300 cursor-default leading-5 rounded-md" type="button" data-page="{{ $filteredData->currentPage() - 1 }}">Previous</button>
<button id="next_button" class="next_button relative inline-flex items-center px-4 py-2 ml-3 text-sm font-medium text-gray-700 bg-white border border-gray-300 leading-5 rounded-md hover:text-gray-500 focus:outline-none focus:ring ring-gray-300 focus:border-blue-300 active:bg-gray-100 active:text-gray-700 transition ease-in-out duration-150" type="button" data-page="{{ $filteredData->currentPage() + 1 }}">Next</button>
</div>
Step 2:- JavaScript Part
<script type="text/javascript">
$(document).ready(function() {
var currentPage = parseInt('{{ $filteredData->currentPage() }}'); // Get the current page from the server-side variable
$('#filter_by_socialsite').on('change', function() {
var filter_by_socialsite = $(this).val();
console.log(filter_by_socialsite);
currentPage = 1; // Reset the current page to 1 when the filter changes
fetch_social_data(filter_by_socialsite, currentPage);
updateURL(currentPage);
});
$('#filter_by_socialsite').select2({
tags: true,
tokenSeparators: [',', ' '],
placeholder: 'Select By Social Site',
width: '100%',
}).on('select2:close', function(e) {
var selectedValue = $(this).val(); // Get the selected value
if (selectedValue === null || selectedValue.length === 0) {
location.reload(); // Refresh the page if the selected value is null or blank
}
}).on('select2:unselect', function(e) {
var selectedValue = $(this).val(); // Get the selected value
if (selectedValue === null || selectedValue.length === 0) {
location.reload(); // Refresh the page if the selected value is null or blank
}
});
$(document).on('click', '.previous_button', function(e) {
e.preventDefault();
var filter_by_socialsite = $('#filter_by_socialsite').val();
if (currentPage > 1) {
currentPage--; // Decrement the current page by 1 if it's greater than 1
fetch_social_data(filter_by_socialsite, currentPage);
updateURL(currentPage);
}
});
$(document).on('click', '.next_button', function(e) {
e.preventDefault();
var filter_by_socialsite = $('#filter_by_socialsite').val();
currentPage++; // Increment the current page by 1
fetch_social_data(filter_by_socialsite, currentPage);
updateURL(currentPage);
});
function fetch_social_data(filter_by_socialsite, page, search_query) {
console.log('fetch_social_data function is called');
$('#user_pic_file').html('');
var cleanPage = page.toString().replace('amp;', '');
$.ajax({
url: "/welcome_influencersocialsite",
method: "GET",
data: {
filter_by_socialsite: filter_by_socialsite.join(','),
page: cleanPage,
search_query: search_query // Include the search query parameter
},
success: function(data) {
console.log(data + ' - Data in success function');
$('#user_pic_file').html('');
$('#user_pic_file').html(data);
var dataCount = $('#count-data').find('.data-item').length; // Update dataCount based on the length of the received data
togglePaginationButtons(dataCount); // Call the function to toggle pagination buttons visibility and pass dataCount
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('AJAX request failed: ' + textStatus, errorThrown);
}
});
}
$('#search_query').on('input', function() {
var searchQuery = $('#search_query').val(); // Get the value of your search input field
if (searchQuery) {
$('.pagination-buttons').show(); // Show the pagination buttons if there is a search query
} else {
$('.pagination-buttons').hide(); // Hide the pagination buttons if there is no search query
}
});
function updateURL(page) {
var newURL = window.location.href.split('?')[0] + '?page=' + page;
window.history.replaceState({}, '', newURL);
}
function togglePaginationButtons(dataCount) {
var searchQuery = $('#search_query').val(); // Get the value of your search input field
var selectedSocialSites = $('#filter_by_socialsite').val(); // Get the selected social sites
var currentPage = parseInt('{{ $filteredData->currentPage() }}'); // Get the current page from the server-side variable
var totalPages = parseInt('{{ $filteredData->lastPage() }}'); // Get the total number of pages from the server-side variable
// Hide the "Previous" button on the first page
if (currentPage === 1) {
$('.previous_button').hide();
} else {
$('.previous_button').show();
}
// Show or hide the "Next" button based on the current page and total pages
if (currentPage >= totalPages || dataCount <= 1) {
$('.next_button').hide(); // Hide the "Next" button if it's the last page or if there are 1 or fewer data
} else if (searchQuery || currentPage < totalPages) {
$('.next_button').show(); // Show the "Next" button if there is a search query or if the current page is less than the total pages
}
// Show or hide the "Previous" button based on the current page and total pages
if (currentPage === totalPages || dataCount <= 1) {
$('.previous_button').show(); // Show the "Previous" button if it's the last page or if there are 1 or fewer data
} else {
$('.previous_button').hide(); // Hide the "Previous" button if it's not the last page
}
}
// Call togglePaginationButtons initially
togglePaginationButtons();
});
</script>
Result:-
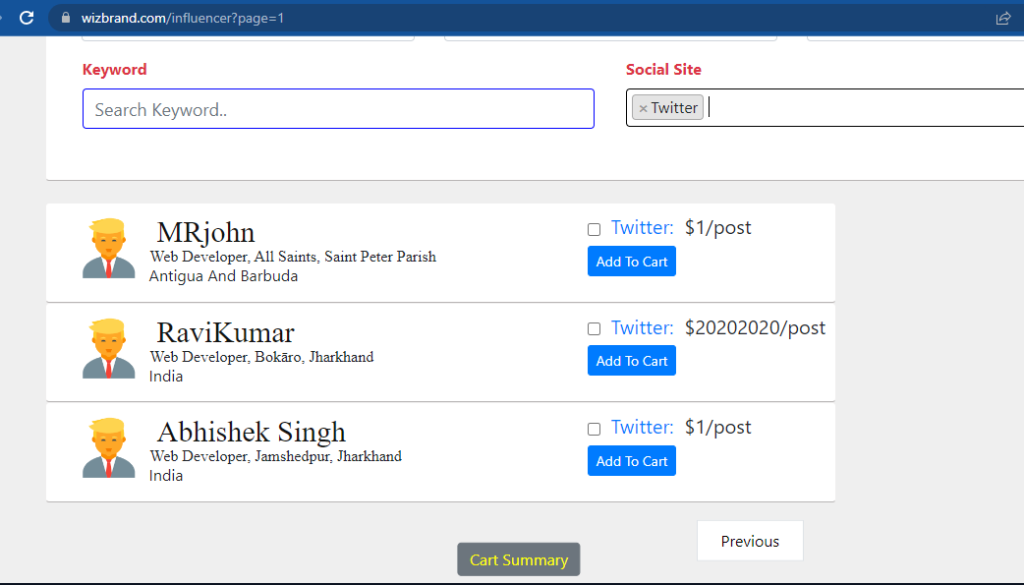