Introduction
Have you ever wondered how to extract the values of the selected checkboxes from a checkboxlist using JQuery? Well, look no further! In this article, we will explore the step-by-step process of achieving this task.
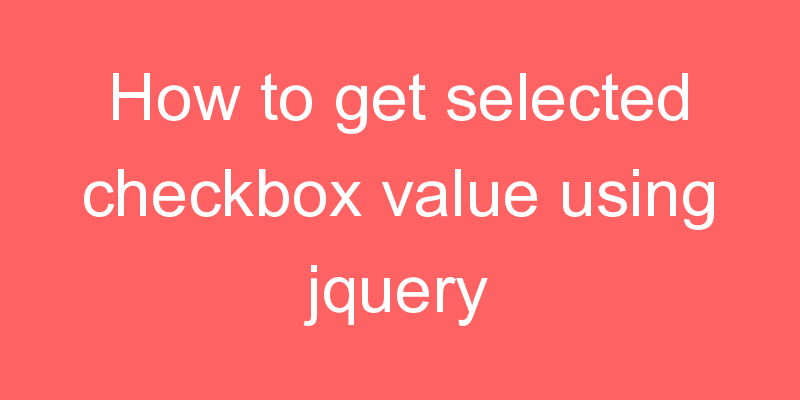
Step: Create a html page
<!DOCTYPE html>
<html>
<head>
<title>Get selected checkbox value from checkboxlist in Jquery - Wizbrand</title>
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
</head>
<body>
<table id="tblPosts">
<tr>
<td><input id="post1" type="checkbox" value="1"/><label for="post1">Roshan</label></td>
</tr>
<tr>
<td><input id="post2" type="checkbox" value="2"/><label for="post2">Amit</label></td>
</tr>
<tr>
<td><input id="post3" type="checkbox" value="3"/><label for="post3">Rakesh</label></td>
</tr>
<tr>
<td><input id="post3" type="checkbox" value="4"/><label for="post3">Laravel Import Export</label></td>
</tr>
<tr>
<td><input id="post4" type="checkbox" value="5"/><label for="post4">Laravel Admin Panel</label></td>
</tr>
</table>
<br />
<input type="button" id="btnClick" value="Get" />
</body>
<script type="text/javascript">
$(function () {
$("#btnClick").click(function () {
var selected = new Array();
$("#tblPosts input[type=checkbox]:checked").each(function () {
selected.push(this.value);
});
if (selected.length > 0) {
alert("Selected values: " + selected.join(","));
}
});
});
</script>
</html>
View:-
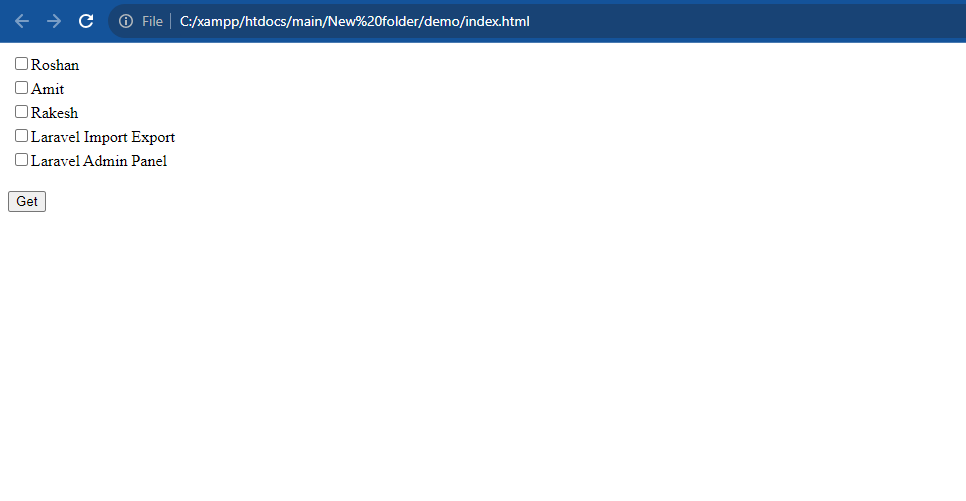
Output:-
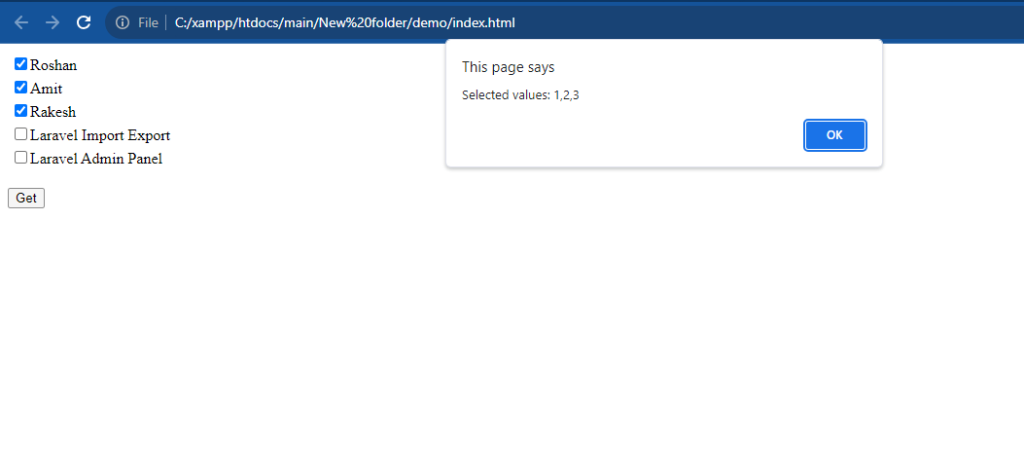
Hopefully, It will help you …!!!