To check the execution time of a query in Laravel, you can make use of Laravel’s built-in query logging and timing features. Here’s how you can do it:
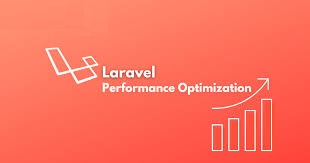
Enable Query Logging:
By default, Laravel logs all queries executed during a request. To enable query logging, you can add the following line to the boot()
method of your AppServiceProvider
class or any other service provider:
use Illuminate\Support\Facades\DB;
// ...
public function boot()
{
DB::connection()->enableQueryLog();
}
Execute Your Query:
Run the query you want to measure the execution time for using Laravel’s query builder or Eloquent ORM. For example:
$users = DB::table('users')->get();
Retrieve Query Execution Time:
After executing the query, you can retrieve the query execution time by accessing the query log and calculating the time difference. You can place the following code after executing the query:
$queryLog = DB::getQueryLog();
$lastQuery = end($queryLog);
$executionTime = $lastQuery['time'];
The $executionTime
variable will contain the execution time of the last executed query in milliseconds.
Display or Log the Execution Time:
You can display the execution time on the page or log it to the Laravel log file for further analysis. For example:
UserController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class UserController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index(Request $request)
{
$startTime = microtime(true);
$users = User::get();
$endTime = microtime(true);
$executionTime = $endTime - $startTime;
dd("Query took " . $executionTime . " seconds to execute.");
}
}
Output:
Query took 0.0032229423555949 seconds to execute.