Introduction
In this article, we will explore the process of using jQuery to validate an empty input field. We will provide a step-by-step guide with a live example to help you understand the concept better.
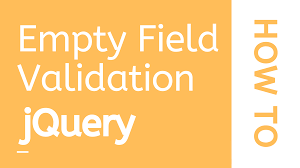
Why is Input Field Validation Important?
Input field validation is crucial in web development as it ensures that users provide the required information before submitting a form. By validating an empty input field, we can prompt users to enter the necessary data, improving the overall user experience.
Step 1: Setting Up the HTML Structure
To begin, we need to set up the HTML structure for our form. Here’s an example of a simple form with an input field:
<form>
<label for="title">Title:</label>
<input type="text" id="title" name="title" required>
<button type="submit">Submit</button>
</form>
In this example, the input field has the required
attribute, which ensures that the field cannot be submitted empty.
Step 2: Including jQuery
Next, we need to include the jQuery library in our HTML file. You can either download the library and host it locally or use a CDN (Content Delivery Network) to include it. Here’s an example of including jQuery using a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 3: Writing the jQuery Code
Now, let’s write the jQuery code to validate the empty input field. We will use the submit()
function to capture the form submission event and perform the validation.
$(document).ready(function() {
$("form").submit(function(event) {
var inputVal = $("#title").val();
if (inputVal === "") {
alert("Please Fill Required Field.");
event.preventDefault();
}
});
});
In this code, we select the form element using the $("form")
selector and attach a submit()
event listener to it. When the form is submitted, we retrieve the value of the input field using the val()
function and check if it is empty. If it is empty, we display an alert message and prevent the form from being submitted using event.preventDefault()
.
Step 4: Testing the Validation
To test the validation, enter a name in the input field and click the submit button. The form should submit successfully. If you try to submit the form without entering a name, an alert message will be displayed, and the form submission will be prevented.
Output:-
