In this tutorial im going to learn how to login and signup with gmail, google account. Please follow some easy steps define below. After follow this tutorial you able to logIn with google.

composer create-project laravel/laravel laravel-amit "5.8.*"
Step 2-> Go to .env and set up connect with database
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravelamit
DB_USERNAME=root
DB_PASSWORD=
3rd step migrate table
php artisan migrate
Let’s go to create authentication and run below command
php artisan make:auth
go to composer.json and paste this version
“laravel/socialite”:”4.4.1″,
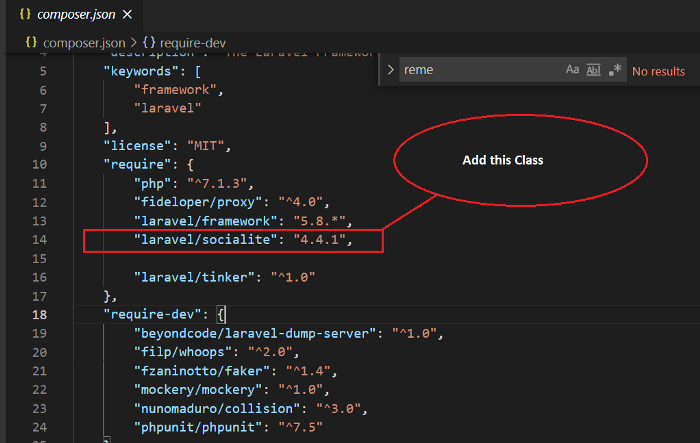
Now update composer
composer update
add provider in config/app.php file
Laravel\Socialite\SocialiteServiceProvider::class,

add alias in config/app.php
'socialite' => Laravel\Socialite\Facades\Socialite::class,

Next create google App credentials here
if you don’t have google app account then you can create from here : Google Developers Console.




Now OAuth client created successfully.

Go to services.php and put your client id and client secret and callback url
'google' => [
'client_id' => '558885374981-aj5n7na3sfntdn7okl2vmu97ka7oqrop.apps.googleusercontent.com',
'client_secret' => 'lI_bzYYrDuOJnLypYbcUcjXp',
'redirect' => 'http://wz-account-admin-ms.co/auth/google/callback',
],

php artisan make:model SocialProvider -m
customize migration social_providers
$table->increments('id');
$table->integer('user_id')->unsigned()->references('id')->on('users');
$table->string('provider_id');
$table->string('provider');
$table->timestamps();

php artisan migrate
add socialProviders() function in user model
go to register controller and paste this code
<?phpnamespace App\Http\Controllers\Auth;use App\User;
use Socialite;
use App\SocialProvider;
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
use Illuminate\Foundation\Auth\RegistersUsers;class RegisterController extends Controller
{
/*
|--------------------------------------------------------------------------
| Register Controller
|--------------------------------------------------------------------------
|
| This controller handles the registration of new users as well as their
| validation and creation. By default this controller uses a trait to
| provide this functionality without requiring any additional code.
|
*/ use RegistersUsers; /**
* Where to redirect users after registration.
*
* @var string
*/
protected $redirectTo = '/home'; /**
* Create a new controller instance.
*
* @return void
*/
public function __construct()
{
$this->middleware('guest');
} /**
* Get a validator for an incoming registration request.
*
* @param array $data
* @return \Illuminate\Contracts\Validation\Validator
*/
protected function validator(array $data)
{
return Validator::make($data, [
'name' => ['required', 'string', 'max:255'],
'email' => ['required', 'string', 'email', 'max:255', 'unique:users'],
'password' => ['required', 'string', 'min:8', 'confirmed'],
]);
} /**
* Create a new user instance after a valid registration.
*
* @param array $data
* @return \App\User
*/
protected function create(array $data)
{
return User::create([
'name' => $data['name'],
'email' => $data['email'],
'password' => Hash::make($data['password']),
]);
} public function redirectToProvider()
{
return Socialite::driver('google')->redirect();
}
// add handleProviderCallback() function in RegisterController
public function handleProviderCallback()
{
try
{
$socialUser = Socialite::driver('google')->user();
}
catch(\Exception $e)
{
return redirect('/');
}
//check if we have logged provider
$socialProvider = SocialProvider::where('provider_id',$socialUser->getId())->first();
if(!$socialProvider)
{
//create a new user and provider
$user = User::firstOrCreate(
['email' => $socialUser->getEmail()],
['name' => $socialUser->getName()]
);
$user->socialProviders()->create(
['provider_id' => $socialUser->getId(), 'provider' => 'google']
);
}
else
$user = $socialProvider->user;
auth()->login($user);
return redirect('/home');
}
}
add routes in web.php
Auth::routes();Route::get('/home', 'HomeController@index')->name('home');Route::get('auth/google', 'Auth\RegisterController@redirectToProvider');
Route::get('auth/google/callback', 'Auth\RegisterController@handleProviderCallback');
Lets got to User.php and add this function below
function socialProviders()
{
return $this->hasMany(SocialProvider::class);
}

Next go to SocialProvider.php and this function
protected $fillable = ['provider_id','provider'];
function user()
{
return $this->belongsTo(User::class);
}

Add Login with google Button On Blade File
<button class="btn btn-primary">
<a class="text-white" href="{{ url('auth/google')}}">Google Login</a>
</button>

Now register page look like this and continue with google

Now continue with google

Now Logged in Successfully…..
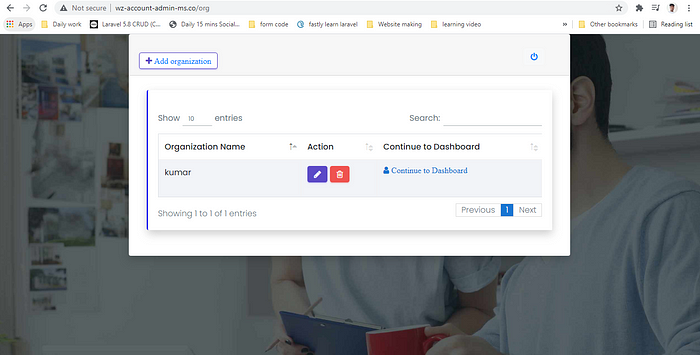
I hope this tutorial is very helpful for you, if code is not working properway please comment below thanks for reach out. ??