In Laravel, the limit()
and offset()
functions are used in conjunction to implement pagination and control the number of results returned from a database query.
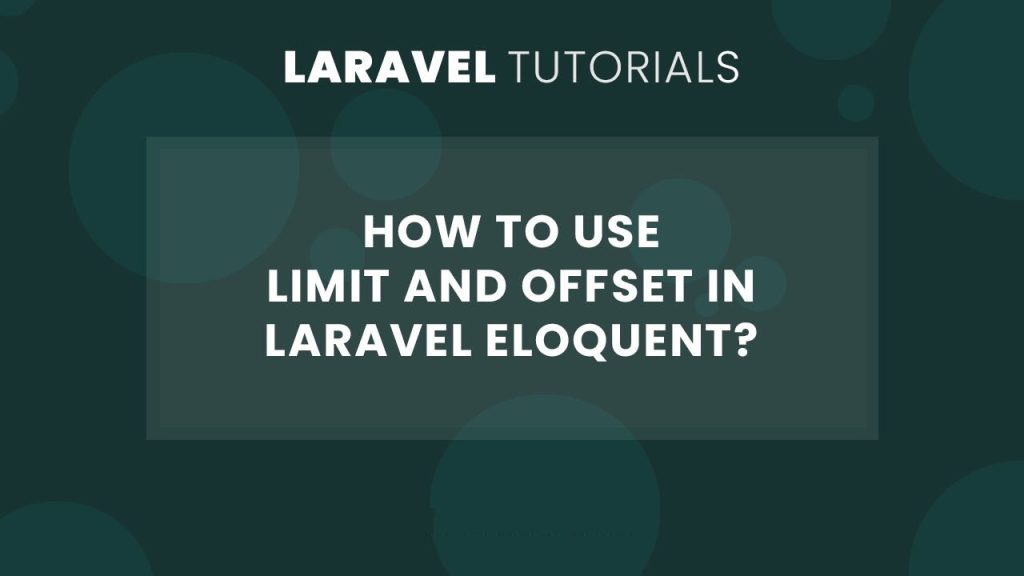
limit()
Function:
- The
limit()
function is used to specify the maximum number of records to be retrieved from the database query. - Syntax:
->limit($value)
- Example:
->limit(10)
– This will limit the result set to 10 records.
offset()
Function:
- The
offset()
function is used to specify the starting point or the number of records to skip from the beginning of the result set. - Syntax:
->offset($value)
- Example:
->offset(5)
– This will skip the first 5 records from the result set.
Function and Uses:
- Pagination: By using the
limit()
andoffset()
functions together, you can implement pagination in Laravel. For example, if you have 100 records and want to display 10 records per page, you can use->limit(10)->offset(0)
for the first page,->limit(10)->offset(10)
for the second page, and so on. This allows you to fetch and display data in smaller chunks, improving performance and user experience. - Limiting Result Set: The
limit()
function is used to restrict the number of records returned by a query. It is commonly used to fetch a specific number of records, such as retrieving the top 5 most recent articles or fetching a limited number of results for a search query. - Offsetting Result Set: The
offset()
function is used to skip a certain number of records from the beginning of the result set. This is useful when you want to retrieve results starting from a particular point, such as fetching records for a specific page or implementing infinite scrolling. - Dynamic Queries: Both
limit()
andoffset()
functions can be used dynamically, allowing you to modify the number of records and the starting point based on user input or application logic. This flexibility enables you to handle various scenarios, such as adjusting the pagination size or implementing custom result set navigation.
By using the limit()
and offset()
functions, you can efficiently control the number of records retrieved from the database and implement pagination in your Laravel application, providing an optimized and user-friendly way to navigate through large data sets.