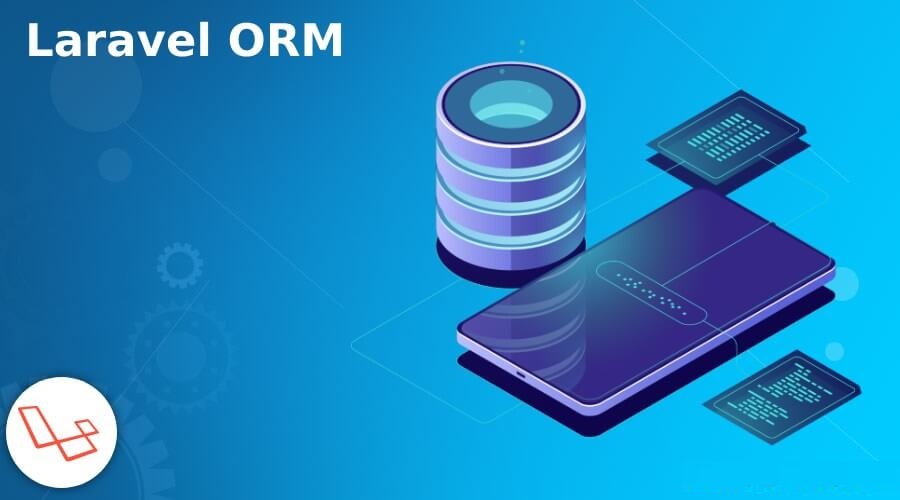
ORM stands for Object-Relational Mapping. It is a technique or a programming paradigm that allows developers to interact with databases using object-oriented programming languages. ORM bridges the gap between the object-oriented world of programming and the relational world of databases.
The primary function of an ORM is to abstract away the complexities of database operations and provide a convenient and intuitive way to perform database CRUD (Create, Read, Update, Delete) operations using objects and classes.
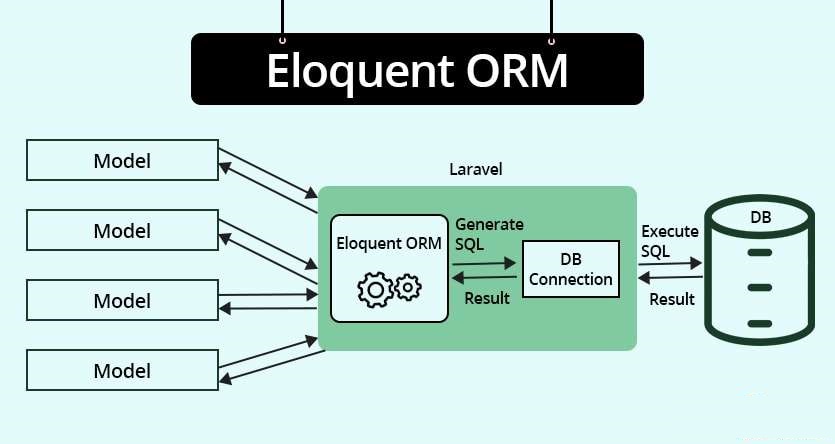
Here is an example of how an ORM can be used in code:
- Define a Model: In an ORM, you typically define a model class that represents a database table. Each property of the class corresponds to a column in the table.
class User extends Model
{
protected $table = 'users';
}
2. Create a Record (Create):
$user = new User;
$user->name = 'Roshan Kumar Jha';
$user->email = 'roshan@example.com';
$user->save();
3. Retrieve Records (Read):
$users = User::all(); // Retrieves all records from the "users" table
4. Update a Record (Update):
$user = User::find(1); // Retrieves a user record with ID 1
$user->name = 'Roshan Kumar Jha';
$user->save();
5. Delete a Record (Delete):
$user = User::find(1);
$user->delete();
In the above example, the ORM handles the translation of object-oriented code to SQL queries, database connections, and result sets. It allows you to work with the database using familiar object-oriented syntax and concepts, eliminating the need to write raw SQL queries manually.
ORMs also provide additional features like eager loading, relationships (such as one-to-many, and many-to-many), query builders, and data validation, making database interactions more efficient and convenient.
Popular ORM libraries for PHP include Eloquent (used in Laravel), Doctrine, and Propel. These libraries provide comprehensive ORM functionalities and are widely used in PHP web development.