Introduction
In this blog post, we will explore how to use jQuery to prevent form submission when the Enter key is pressed. We will specifically focus on excluding the Enter key functionality for the textarea element. So, let’s dive in!
Why Prevent Submit on Enter Key?
When users fill out forms on websites, they often use the Enter key to move between input fields. However, in some cases, pressing Enter unintentionally triggers the form submission, causing frustrations for users. By preventing form submission on Enter key press, we can enhance the user experience and prevent accidental submissions.
The jQuery Solution
$(document).ready(function() {
$(window).keydown(function(event){
if((event.keyCode == 13) && ($(event.target)[0]!=$("textarea")[0])) {
event.preventDefault();
return false;
}
});
});
index.html
<!DOCTYPE html>
<html>
<head>
<title>JQuery prevent to enter to submit except Textarea</title>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.js"></script>
</head>
<body>
<form method="POST">
<strong>Name:</strong>
<input type="text" name="name" placeholder="Name">
<strong>Details:</strong>
<textarea></textarea>
<button type="submit">Submit</button>
</form>
<script type="text/javascript">
$(document).ready(function() {
$(window).keydown(function(event) {
// Check if the pressed key is Enter and the focused element is not a textarea
if (event.keyCode === 13 && $(event.target)[0].tagName !== 'TEXTAREA') {
event.preventDefault();
alert('Form submission prevented. Enter key is disabled outside of textarea.');
return false;
}
});
});
</script>
</body>
</html>
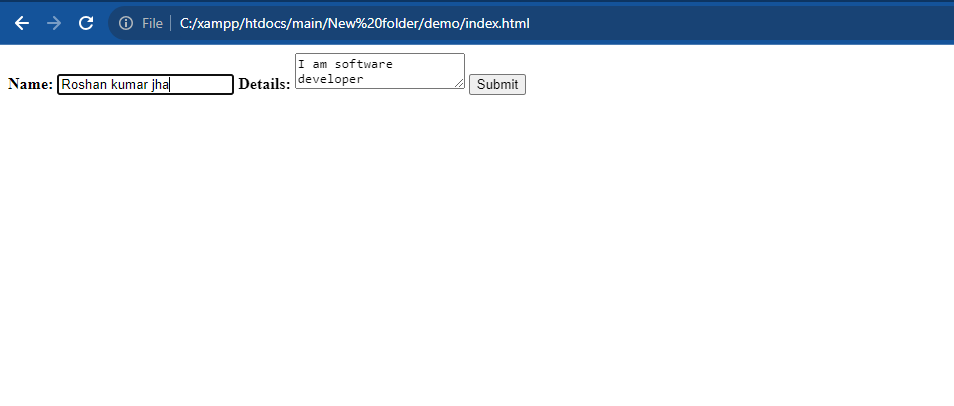
Hopefully, It will help you!!!