When working with Laravel, understanding the concept of the $this
keyword within controllers is crucial for building robust and maintainable applications. In this article, we’ll delve into the importance of $this
in Laravel controllers and explore how it facilitates interaction with various components of the framework.
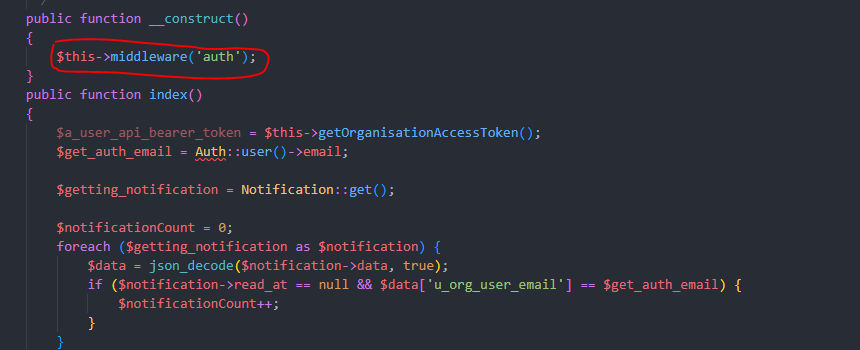
What is $this
in Laravel?
In object-oriented programming, $this
refers to the current instance of the class. In the context of Laravel controllers, $this
is used to access properties and methods within the controller itself, as well as to interact with other Laravel components such as services, models, views, and middleware.
Accessing Properties and Methods
Let’s start by looking at how $this
is used to access properties and methods within a Laravel controller:
<?php
namespace App\Http\Controllers;
use App\Models\User;
use Illuminate\Http\Request;
class UserController extends Controller
{
private $userRepository;
public function __construct(User $userRepository)
{
$this->userRepository = $userRepository;
}
public function index()
{
$users = $this->userRepository->getAllUsers();
return view('users.index', compact('users'));
}
}
In this example, $this->userRepository
accesses the userRepository
property defined within the controller. We assign an instance of the User
model to this property via constructor injection
Interacting with Laravel Components
Laravel controllers often need to interact with other framework components such as models, services, and views. $this
provides a convenient way to access these components within the controller:
public function show($id)
{
$user = $this->userRepository->find($id);
return view('users.show', compact('user'));
}
Here, $this->userRepository->find($id)
fetches a user record from the database using the User
model. We then pass this data to the users.show
view for display.