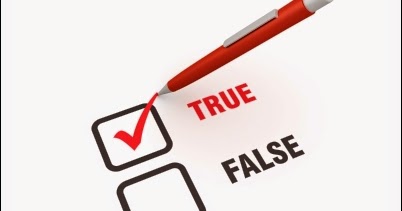
This article details the definition of a Boolean data type and explains its use in programming languages, also examples of Boolean operators that will be useful when understanding Boolean logic and conditional statements.
Mainly there are 3 types of data: text, numbers and Booleans. A Boolean data type is a value that can only be either true or false. A “true” Boolean value is that the object is valid (e.g. an email address has been typed correctly). A “false” Boolean value indicates that the object is invalid and has not been done correctly (e.g. you’ve forgotten to fill out a required field). Boolean values have two possible states: true and false. In binary, these are represented by 1 and 0.
Boolean algebra is a type of math that deals with operations on logical values, including binary variables. It is the foundation for decisions in programs, so it’s important to understand how Booleans work.
Truth and false values
There are some special values in programming languages which can be treated as both text and Booleans. These are known as “truth” or “false” values, depending on whether they evaluate to true or false respectively. For example, 0 is a false value because it evaluates to false but “0” is a truth value as a defined string.
Boolean value operators
The following are examples of the Boolean value operators in programming:
- >= – True if a number is greater than or equal to another.
- <= – True if a number is less than or equal to another.
- == – True if two values are equivalent.
- != – True if two values are not equivalent.
- && – True if both values are true.
- || – True if either of the values are true.
- ! – True if the value is false.
- ~ – Reverses all of the bits in a variable
Boolean operators are used to make decisions in programs and indicate how the program should behave. For example, if p is true AND q is also true, then do something.
Boolean use-case example
Boolean values are used in conditional tests as discussed below.
- Checks that the email address is valid.
- Checks that the password is at least 6 characters long.
- Checks that both fields are filled out correctly.
Example:-
In this example we have to show that 40 > 19,
Output:-
JavaScript Booleans
true
What are some programming languages that support Boolean data types?
Booleans are available in most programming languages. If you’re using JavaScript, Java, PHP, Python, C, C++ or Swift then you should have access to the Boolean data type.
Summary
In this blog post you learned what Boolean values are and how they differ from other types of data. You also learned why it’s important to understand how Booleans work, and how to use Boolean operators in programming.
I hope you like this information about Boolean helpful.
Thank You!!