JSON (JavaScript Object Notation) is a lightweight data interchange format that is widely used for transmitting data between a server and a web application. In JavaScript, the JSON.stringify()
and JSON.parse()
methods play important roles in converting JavaScript objects to JSON strings and vice versa. In this blog post, we will explore these methods in detail and demonstrate their usage with examples.
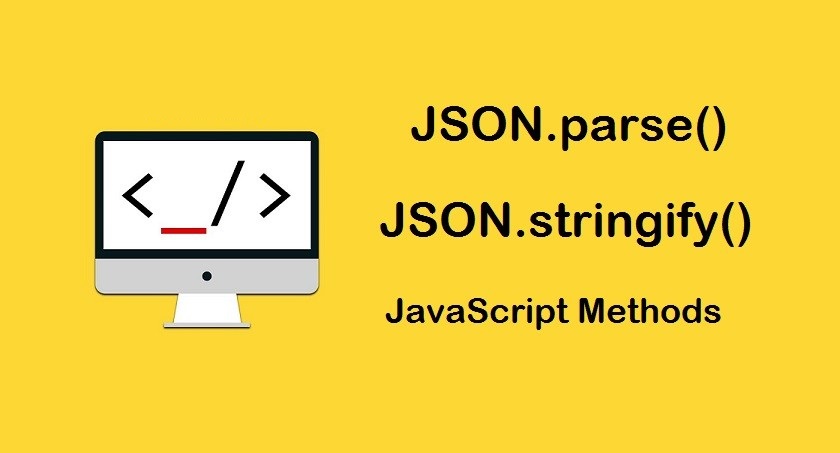
Understanding JSON.stringify():
The JSON.stringify()
method converts a JavaScript object or value to a JSON string. This method takes an object as its parameter and returns a JSON string representation of that object.
const obj = { name: 'Roshan', age: 28, city: 'Bokaro' };
const jsonString = JSON.stringify(obj);
console.log(jsonString);
// Output: {"name":"Roshan","age":28,"city":"Bokaro"}
Understanding JSON.parse():
The JSON.parse()
method parses a JSON string and returns a JavaScript object. This method takes a JSON string as its parameter and returns a JavaScript object representation of that string.
const jsonString = '{"name":"Roshan","age":28,"city":"Bokaro"}';
const obj = JSON.parse(jsonString);
console.log(obj);
// Output: { name: 'Roshan', age: 28, city: 'Bokaro' }
Combining JSON.stringify() and JSON.parse():
One common use case for JSON.stringify()
and JSON.parse()
is to serialize and deserialize JavaScript objects, especially when transmitting data between a client and a server.
const obj = { name: 'Roshan', age: 28, city: 'Bokaro' };
const jsonString = JSON.stringify(obj);
// Deserialize: Convert JSON string back to JavaScript object
const newObj = JSON.parse(jsonString);
console.log(newObj);
// Output: { name: 'Roshan', age: 28, city: 'Bokaro' }
Conclusion:
The JSON.stringify()
and JSON.parse()
methods are powerful tools in JavaScript for converting between JavaScript objects and JSON strings. Understanding how to use these methods effectively is essential for working with JSON data in web development projects. By mastering these methods, developers can seamlessly serialize and deserialize data, facilitating smooth communication between client-side and server-side components of web applications.